Context Selector Widget
This widget is a standardized context selection UI, suitable to be dropped in when a Toolkit App needs a user to select a context:
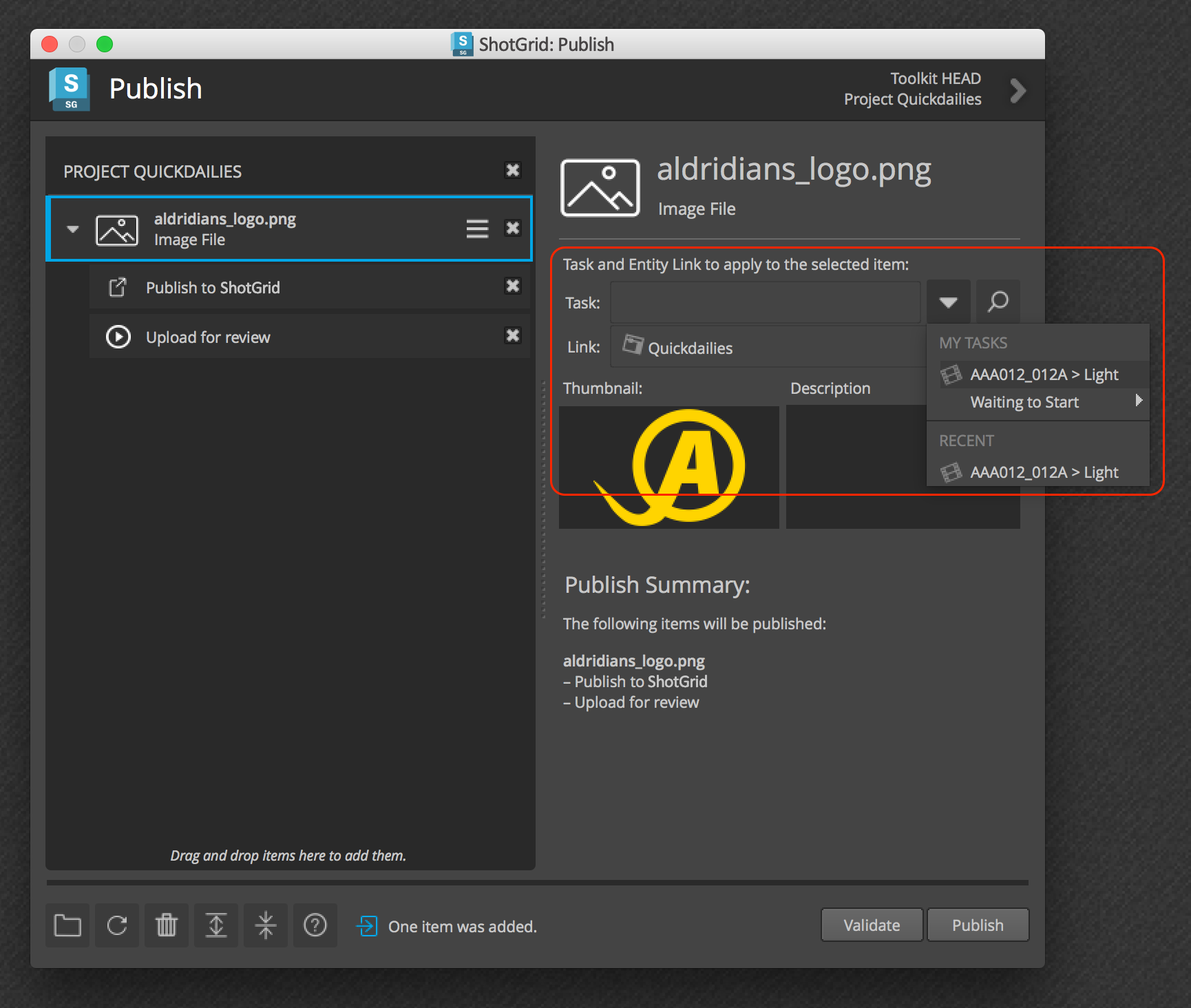
The widget includes the following features:
Uses standard auto completers to allow a user to quickly find tasks or links.
Keeps recently selected tasks on the menu for quick lookup.
Shows related tasks for a given task or link.
Configurable to be just a viewer rather than a selector.
Configurable title
- class context_selector.ContextWidget(parent)[source]
Widget which represents the current context and allows the user to search for a different context via search completer. A menu is also provided for recent contexts as well as tasks assigned to the user.
- Signal context_changed(context_obj):
Fires when when the user selects a context.
- Parameters:
parent (
QObject
) – The model parent.
- save_recent_contexts()[source]
Should be called by the parent widget, typically when the dialog closes, to ensure the recent contexts are saved to disk when closing.
- set_context(context, task_display_override=None, link_display_override=None)[source]
Set the context to display in the widget.
The initial display values can be overridden via the task and link override args.
- set_up(task_manager)[source]
Handles initial set up of the widget. Includes setting up menu, running any background set up tasks, etc.
- Parameters:
task_manager (
BackgroundTaskManager
) – Background task manager to use
- restrict_entity_types_by_link(entity_type, field_name)[source]
Specify what entries should show up in the list of links when using the auto completer.
For the simple case where you just want to show a given set of entity types, use
restrict_entity_types()
. This method is a more complex restriction suitable for workflows around publishing and review.This method will look at the given link field (e.g.
PublishedFile.entity
) and inspect the shotgun schema to see which entity types are valid connections to this field (e.g. in this example which entity types can you can associate a publish with) and those types will appear in the list of items shown by the auto completer.This is useful when you want to use the context widget in conjunction with workflows related to for example publishes, versions or notes and you want to restrict the entities displayed by the auto completer to the ones that have been configured in the shotgun site schema to be able to associate with the given type.
- restrict_entity_types(entity_types)[source]
Restrict which entity types should show up in the list of matches.
- Parameters:
entity_types (list) – List of entity types
- property context_label
The label for the context widget.
- set_task_tooltip(tooltip)[source]
Specify a string (can be html) which should be shown as the tooltip for the task selection widget
- Parameters:
tooltip (str) – Tooltip plaintext or html
- set_link_tooltip(tooltip)[source]
Specify a string (can be html) which should be shown as the tooltip for the link selection widget
- Parameters:
tooltip (str) – Tooltip plaintext or html
- enable_editing(enabled, message=None)[source]
Show/hide the input widgets and display a message in the context label.
- Parameters:
enabled (bool) – Indicates if task/link selectors should be shown
message (str) – Message to display on
context_label()
- backgroundRole(self) PySide2.QtGui.QPalette.ColorRole
- backingStore(self) PySide2.QtGui.QBackingStore
- baseSize(self) PySide2.QtCore.QSize
- childAt(self, p: PySide2.QtCore.QPoint) PySide2.QtWidgets.QWidget
- childAt(self, x: int, y: int) PySide2.QtWidgets.QWidget
- childrenRect(self) PySide2.QtCore.QRect
- childrenRegion(self) PySide2.QtGui.QRegion
- static connect(arg__1: PySide2.QtCore.QObject, arg__2: bytes, arg__3: Callable, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) bool
- static connect(self, arg__1: bytes, arg__2: Callable, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) bool
- static connect(self, arg__1: bytes, arg__2: PySide2.QtCore.QObject, arg__3: bytes, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) bool
- static connect(self, sender: PySide2.QtCore.QObject, signal: bytes, member: bytes, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) PySide2.QtCore.QMetaObject.Connection
- static connect(sender: PySide2.QtCore.QObject, signal: PySide2.QtCore.QMetaMethod, receiver: PySide2.QtCore.QObject, method: PySide2.QtCore.QMetaMethod, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) PySide2.QtCore.QMetaObject.Connection
- static connect(sender: PySide2.QtCore.QObject, signal: bytes, receiver: PySide2.QtCore.QObject, member: bytes, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) PySide2.QtCore.QMetaObject.Connection
- contentsMargins(self) PySide2.QtCore.QMargins
- contentsRect(self) PySide2.QtCore.QRect
- contextMenuPolicy(self) PySide2.QtCore.Qt.ContextMenuPolicy
- static createWindowContainer(window: PySide2.QtGui.QWindow, parent: Optional[PySide2.QtWidgets.QWidget] = None, flags: PySide2.QtCore.Qt.WindowFlags = Default(Qt.WindowFlags)) PySide2.QtWidgets.QWidget
- cursor(self) PySide2.QtGui.QCursor
- static disconnect(arg__1: PySide2.QtCore.QMetaObject.Connection) bool
- static disconnect(arg__1: PySide2.QtCore.QObject, arg__2: bytes, arg__3: Callable) bool
- static disconnect(self, arg__1: bytes, arg__2: Callable) bool
- static disconnect(self, receiver: PySide2.QtCore.QObject, member: Optional[bytes] = None) bool
- static disconnect(self, signal: bytes, receiver: PySide2.QtCore.QObject, member: bytes) bool
- static disconnect(sender: PySide2.QtCore.QObject, signal: PySide2.QtCore.QMetaMethod, receiver: PySide2.QtCore.QObject, member: PySide2.QtCore.QMetaMethod) bool
- static disconnect(sender: PySide2.QtCore.QObject, signal: bytes, receiver: PySide2.QtCore.QObject, member: bytes) bool
- findChildren(self, arg__1: type, arg__2: PySide2.QtCore.QRegExp) Iterable
- findChildren(self, arg__1: type, arg__2: PySide2.QtCore.QRegularExpression) Iterable
- findChildren(self, arg__1: type, arg__2: str = '') Iterable
- focusPolicy(self) PySide2.QtCore.Qt.FocusPolicy
- focusProxy(self) PySide2.QtWidgets.QWidget
- focusWidget(self) PySide2.QtWidgets.QWidget
- font(self) PySide2.QtGui.QFont
- fontInfo(self) PySide2.QtGui.QFontInfo
- fontMetrics(self) PySide2.QtGui.QFontMetrics
- foregroundRole(self) PySide2.QtGui.QPalette.ColorRole
- frameGeometry(self) PySide2.QtCore.QRect
- frameSize(self) PySide2.QtCore.QSize
- geometry(self) PySide2.QtCore.QRect
- grab(self, rectangle: PySide2.QtCore.QRect = PySide2.QtCore.QRect(0, 0, -1, -1)) PySide2.QtGui.QPixmap
- grabGesture(self, type: PySide2.QtCore.Qt.GestureType, flags: PySide2.QtCore.Qt.GestureFlags = Default(Qt.GestureFlags)) None
- grabShortcut(self, key: PySide2.QtGui.QKeySequence, context: PySide2.QtCore.Qt.ShortcutContext = PySide2.QtCore.Qt.ShortcutContext.WindowShortcut) int
- graphicsEffect(self) PySide2.QtWidgets.QGraphicsEffect
- graphicsProxyWidget(self) PySide2.QtWidgets.QGraphicsProxyWidget
- inputMethodHints(self) PySide2.QtCore.Qt.InputMethodHints
- insertActions(self, before: PySide2.QtWidgets.QAction, actions: Sequence[PySide2.QtWidgets.QAction]) None
- static keyboardGrabber() PySide2.QtWidgets.QWidget
- layout(self) PySide2.QtWidgets.QLayout
- layoutDirection(self) PySide2.QtCore.Qt.LayoutDirection
- locale(self) PySide2.QtCore.QLocale
- mapFrom(self, arg__1: PySide2.QtWidgets.QWidget, arg__2: PySide2.QtCore.QPoint) PySide2.QtCore.QPoint
- mapFromGlobal(self, arg__1: PySide2.QtCore.QPoint) PySide2.QtCore.QPoint
- mapFromParent(self, arg__1: PySide2.QtCore.QPoint) PySide2.QtCore.QPoint
- mapTo(self, arg__1: PySide2.QtWidgets.QWidget, arg__2: PySide2.QtCore.QPoint) PySide2.QtCore.QPoint
- mapToGlobal(self, arg__1: PySide2.QtCore.QPoint) PySide2.QtCore.QPoint
- mapToParent(self, arg__1: PySide2.QtCore.QPoint) PySide2.QtCore.QPoint
- mask(self) PySide2.QtGui.QRegion
- maximumSize(self) PySide2.QtCore.QSize
- metaObject(self) PySide2.QtCore.QMetaObject
- minimumSize(self) PySide2.QtCore.QSize
- minimumSizeHint(self) PySide2.QtCore.QSize
- static mouseGrabber() PySide2.QtWidgets.QWidget
- nativeParentWidget(self) PySide2.QtWidgets.QWidget
- nextInFocusChain(self) PySide2.QtWidgets.QWidget
- normalGeometry(self) PySide2.QtCore.QRect
- paintEngine(self) PySide2.QtGui.QPaintEngine
- palette(self) PySide2.QtGui.QPalette
- parent(self) PySide2.QtCore.QObject
- parentWidget(self) PySide2.QtWidgets.QWidget
- pos(self) PySide2.QtCore.QPoint
- previousInFocusChain(self) PySide2.QtWidgets.QWidget
- rect(self) PySide2.QtCore.QRect
- redirected(self, offset: PySide2.QtCore.QPoint) PySide2.QtGui.QPaintDevice
- render(self, painter: PySide2.QtGui.QPainter, targetOffset: PySide2.QtCore.QPoint, sourceRegion: PySide2.QtGui.QRegion = Default(QRegion), renderFlags: PySide2.QtWidgets.QWidget.RenderFlags = Instance(QWidget.RenderFlags(QWidget.DrawWindowBackground | QWidget.DrawChildren))) None
- render(self, target: PySide2.QtGui.QPaintDevice, targetOffset: PySide2.QtCore.QPoint = Default(QPoint), sourceRegion: PySide2.QtGui.QRegion = Default(QRegion), renderFlags: PySide2.QtWidgets.QWidget.RenderFlags = Instance(QWidget.RenderFlags(QWidget.DrawWindowBackground | QWidget.DrawChildren))) None
- repaint(self) None
- repaint(self, arg__1: PySide2.QtCore.QRect) None
- repaint(self, arg__1: PySide2.QtGui.QRegion) None
- repaint(self, x: int, y: int, w: int, h: int) None
- saveGeometry(self) PySide2.QtCore.QByteArray
- screen(self) PySide2.QtGui.QScreen
- scroll(self, dx: int, dy: int) None
- scroll(self, dx: int, dy: int, arg__3: PySide2.QtCore.QRect) None
- sender(self) PySide2.QtCore.QObject
- setBaseSize(self, arg__1: PySide2.QtCore.QSize) None
- setBaseSize(self, basew: int, baseh: int) None
- setContentsMargins(self, left: int, top: int, right: int, bottom: int) None
- setContentsMargins(self, margins: PySide2.QtCore.QMargins) None
- setGeometry(self, arg__1: PySide2.QtCore.QRect) None
- setGeometry(self, x: int, y: int, w: int, h: int) None
- setMask(self, arg__1: PySide2.QtGui.QBitmap) None
- setMask(self, arg__1: PySide2.QtGui.QRegion) None
- setMaximumSize(self, arg__1: PySide2.QtCore.QSize) None
- setMaximumSize(self, maxw: int, maxh: int) None
- setMinimumSize(self, arg__1: PySide2.QtCore.QSize) None
- setMinimumSize(self, minw: int, minh: int) None
- setParent(self, parent: PySide2.QtCore.QObject) None
- setParent(self, parent: PySide2.QtWidgets.QWidget) None
- setParent(self, parent: PySide2.QtWidgets.QWidget, f: PySide2.QtCore.Qt.WindowFlags) None
- setSizeIncrement(self, arg__1: PySide2.QtCore.QSize) None
- setSizeIncrement(self, w: int, h: int) None
- setSizePolicy(self, arg__1: PySide2.QtWidgets.QSizePolicy) None
- setSizePolicy(self, horizontal: PySide2.QtWidgets.QSizePolicy.Policy, vertical: PySide2.QtWidgets.QSizePolicy.Policy) None
- size(self) PySide2.QtCore.QSize
- sizeHint(self) PySide2.QtCore.QSize
- sizeIncrement(self) PySide2.QtCore.QSize
- sizePolicy(self) PySide2.QtWidgets.QSizePolicy
- startTimer(self, interval: int, timerType: PySide2.QtCore.Qt.TimerType = PySide2.QtCore.Qt.TimerType.CoarseTimer) int
- style(self) PySide2.QtWidgets.QStyle
- thread(self) PySide2.QtCore.QThread
- topLevelWidget(self) PySide2.QtWidgets.QWidget
- update(self) None
- update(self, arg__1: PySide2.QtCore.QRect) None
- update(self, arg__1: PySide2.QtGui.QRegion) None
- update(self, x: int, y: int, w: int, h: int) None
- visibleRegion(self) PySide2.QtGui.QRegion
- window(self) PySide2.QtWidgets.QWidget
- windowFlags(self) PySide2.QtCore.Qt.WindowFlags
- windowHandle(self) PySide2.QtGui.QWindow
- windowIcon(self) PySide2.QtGui.QIcon
- windowModality(self) PySide2.QtCore.Qt.WindowModality
- windowState(self) PySide2.QtCore.Qt.WindowStates
- windowType(self) PySide2.QtCore.Qt.WindowType