Text Overlay Widget
Introduction
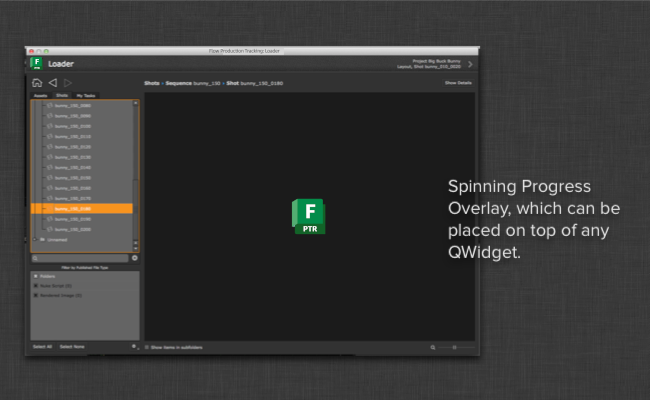
The progress overlay module provides a standardized progress overlay widget which
can easily be placed on top of any other PySide.QtGui.QWidget
to indicate that work is happening
and potentially report messages back to the user. Once you have instantiated and
placed it on top of another widget, you can execute various methods to control its state.
Sample Code
The following sample code shows how to import the overlay module, connect it to a widget and then control the overlay state:
# example of how the overlay can be used within your app code
# import the module - note that this is using the special
# import_framework code so it won't work outside an app
overlay = sgtk.platform.import_framework("tk-framework-qtwidgets", "overlay_widget")
# now inside your app constructor, create an overlay and parent it to something
self._overlay = overlay.ShotgunOverlayWidget(my_widget)
# now you can use the overlay to report things to the user
try:
self._overlay.start_spin()
run_some_code_here()
except Exception, e:
self._overlay.show_error_message("An error was reported: %s" % e)
finally:
self._overlay.hide()
Please note that the example above is crude and for heavy computational work we recommend an asynchronous approach with a worker thread for better UI responsiveness.
ShotgunSpinningWidget
- class overlay_widget.ShotgunSpinningWidget(parent)[source]
Bases:
QWidget
Overlay widget that can be placed on top over any QT widget. Once you have placed the overlay widget, you can use it to display a spinner or report progress in the form of an arc that goes from 0 to 360 degrees.
- Parameters:
parent (
PySide.QtGui.QWidget
) – Widget to attach the overlay to
- start_spin()[source]
Enables the overlay and shows an animated spinner.
If you want to stop the spinning, call
hide()
.
- start_progress()[source]
Enables the overlay and shows an animated progress arc.
If you want to stop the progress, call
hide()
.
ShotgunOverlayWidget
- class overlay_widget.ShotgunOverlayWidget(parent)[source]
Bases:
QLabel
Overlay widget that can be placed on top over any QT widget. Once you have placed the overlay widget, you can use it to display information, errors, a spinner etc.
The
show_message()
andshow_error_message()
accept both regular text and HTML to format the error message.Constants
INFO_COLOR
andERROR_COLOR
are provided on the class as shorthand for the colors employed by theshow_message()
andshow_error_message()
methods.- Parameters:
parent (
PySide.QtGui.QWidget
) – Widget to attach the overlay to
- start_spin()[source]
Enables the overlay and shows an animated spinner.
If you want to stop the spinning, call
hide()
.
- show_error_message(msg)[source]
Enables the overlay and displays an a error message centered in the middle of the overlay.
- Parameters:
msg – Message to display
- show_message(msg)[source]
Display a message centered on the overlay. If an error is already being displayed by the overlay at this point, nothing will happen.
- Parameters:
msg – Message to display
- Returns:
True if message was displayed, False otherwise
- show_message_pixmap(pixmap)[source]
Show an info message in the form of a pixmap. If an error is already being displayed by the overlay, the pixmap will not be shown.
- Parameters:
pixamp – Image to display
- Returns:
True if pixmap was displayed, False otherwise
- hide(hide_errors=True)[source]
Hides the overlay.
- Parameters:
hide_errors – If set to False, errors are not hidden.
- alignment(self) PySide2.QtCore.Qt.Alignment
- backgroundRole(self) PySide2.QtGui.QPalette.ColorRole
- backingStore(self) PySide2.QtGui.QBackingStore
- baseSize(self) PySide2.QtCore.QSize
- buddy(self) PySide2.QtWidgets.QWidget
- childAt(self, p: PySide2.QtCore.QPoint) PySide2.QtWidgets.QWidget
- childAt(self, x: int, y: int) PySide2.QtWidgets.QWidget
- childrenRect(self) PySide2.QtCore.QRect
- childrenRegion(self) PySide2.QtGui.QRegion
- static connect(arg__1: PySide2.QtCore.QObject, arg__2: bytes, arg__3: Callable, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) bool
- static connect(self, arg__1: bytes, arg__2: Callable, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) bool
- static connect(self, arg__1: bytes, arg__2: PySide2.QtCore.QObject, arg__3: bytes, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) bool
- static connect(self, sender: PySide2.QtCore.QObject, signal: bytes, member: bytes, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) PySide2.QtCore.QMetaObject.Connection
- static connect(sender: PySide2.QtCore.QObject, signal: PySide2.QtCore.QMetaMethod, receiver: PySide2.QtCore.QObject, method: PySide2.QtCore.QMetaMethod, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) PySide2.QtCore.QMetaObject.Connection
- static connect(sender: PySide2.QtCore.QObject, signal: bytes, receiver: PySide2.QtCore.QObject, member: bytes, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) PySide2.QtCore.QMetaObject.Connection
- contentsMargins(self) PySide2.QtCore.QMargins
- contentsRect(self) PySide2.QtCore.QRect
- contextMenuPolicy(self) PySide2.QtCore.Qt.ContextMenuPolicy
- static createWindowContainer(window: PySide2.QtGui.QWindow, parent: Optional[PySide2.QtWidgets.QWidget] = None, flags: PySide2.QtCore.Qt.WindowFlags = Default(Qt.WindowFlags)) PySide2.QtWidgets.QWidget
- cursor(self) PySide2.QtGui.QCursor
- static disconnect(arg__1: PySide2.QtCore.QMetaObject.Connection) bool
- static disconnect(arg__1: PySide2.QtCore.QObject, arg__2: bytes, arg__3: Callable) bool
- static disconnect(self, arg__1: bytes, arg__2: Callable) bool
- static disconnect(self, receiver: PySide2.QtCore.QObject, member: Optional[bytes] = None) bool
- static disconnect(self, signal: bytes, receiver: PySide2.QtCore.QObject, member: bytes) bool
- static disconnect(sender: PySide2.QtCore.QObject, signal: PySide2.QtCore.QMetaMethod, receiver: PySide2.QtCore.QObject, member: PySide2.QtCore.QMetaMethod) bool
- static disconnect(sender: PySide2.QtCore.QObject, signal: bytes, receiver: PySide2.QtCore.QObject, member: bytes) bool
- findChildren(self, arg__1: type, arg__2: PySide2.QtCore.QRegExp) Iterable
- findChildren(self, arg__1: type, arg__2: PySide2.QtCore.QRegularExpression) Iterable
- findChildren(self, arg__1: type, arg__2: str = '') Iterable
- focusPolicy(self) PySide2.QtCore.Qt.FocusPolicy
- focusProxy(self) PySide2.QtWidgets.QWidget
- focusWidget(self) PySide2.QtWidgets.QWidget
- font(self) PySide2.QtGui.QFont
- fontInfo(self) PySide2.QtGui.QFontInfo
- fontMetrics(self) PySide2.QtGui.QFontMetrics
- foregroundRole(self) PySide2.QtGui.QPalette.ColorRole
- frameGeometry(self) PySide2.QtCore.QRect
- frameRect(self) PySide2.QtCore.QRect
- frameShadow(self) PySide2.QtWidgets.QFrame.Shadow
- frameShape(self) PySide2.QtWidgets.QFrame.Shape
- frameSize(self) PySide2.QtCore.QSize
- geometry(self) PySide2.QtCore.QRect
- grab(self, rectangle: PySide2.QtCore.QRect = PySide2.QtCore.QRect(0, 0, -1, -1)) PySide2.QtGui.QPixmap
- grabGesture(self, type: PySide2.QtCore.Qt.GestureType, flags: PySide2.QtCore.Qt.GestureFlags = Default(Qt.GestureFlags)) None
- grabShortcut(self, key: PySide2.QtGui.QKeySequence, context: PySide2.QtCore.Qt.ShortcutContext = PySide2.QtCore.Qt.ShortcutContext.WindowShortcut) int
- graphicsEffect(self) PySide2.QtWidgets.QGraphicsEffect
- graphicsProxyWidget(self) PySide2.QtWidgets.QGraphicsProxyWidget
- inputMethodHints(self) PySide2.QtCore.Qt.InputMethodHints
- insertActions(self, before: PySide2.QtWidgets.QAction, actions: Sequence[PySide2.QtWidgets.QAction]) None
- static keyboardGrabber() PySide2.QtWidgets.QWidget
- layout(self) PySide2.QtWidgets.QLayout
- layoutDirection(self) PySide2.QtCore.Qt.LayoutDirection
- locale(self) PySide2.QtCore.QLocale
- mapFrom(self, arg__1: PySide2.QtWidgets.QWidget, arg__2: PySide2.QtCore.QPoint) PySide2.QtCore.QPoint
- mapFromGlobal(self, arg__1: PySide2.QtCore.QPoint) PySide2.QtCore.QPoint
- mapFromParent(self, arg__1: PySide2.QtCore.QPoint) PySide2.QtCore.QPoint
- mapTo(self, arg__1: PySide2.QtWidgets.QWidget, arg__2: PySide2.QtCore.QPoint) PySide2.QtCore.QPoint
- mapToGlobal(self, arg__1: PySide2.QtCore.QPoint) PySide2.QtCore.QPoint
- mapToParent(self, arg__1: PySide2.QtCore.QPoint) PySide2.QtCore.QPoint
- mask(self) PySide2.QtGui.QRegion
- maximumSize(self) PySide2.QtCore.QSize
- metaObject(self) PySide2.QtCore.QMetaObject
- minimumSize(self) PySide2.QtCore.QSize
- minimumSizeHint(self) PySide2.QtCore.QSize
- static mouseGrabber() PySide2.QtWidgets.QWidget
- movie(self) PySide2.QtGui.QMovie
- nativeParentWidget(self) PySide2.QtWidgets.QWidget
- nextInFocusChain(self) PySide2.QtWidgets.QWidget
- normalGeometry(self) PySide2.QtCore.QRect
- paintEngine(self) PySide2.QtGui.QPaintEngine
- palette(self) PySide2.QtGui.QPalette
- parent(self) PySide2.QtCore.QObject
- parentWidget(self) PySide2.QtWidgets.QWidget
- picture(self) PySide2.QtGui.QPicture
- pixmap(self) PySide2.QtGui.QPixmap
- pos(self) PySide2.QtCore.QPoint
- previousInFocusChain(self) PySide2.QtWidgets.QWidget
- rect(self) PySide2.QtCore.QRect
- redirected(self, offset: PySide2.QtCore.QPoint) PySide2.QtGui.QPaintDevice
- render(self, painter: PySide2.QtGui.QPainter, targetOffset: PySide2.QtCore.QPoint, sourceRegion: PySide2.QtGui.QRegion = Default(QRegion), renderFlags: PySide2.QtWidgets.QWidget.RenderFlags = Instance(QWidget.RenderFlags(QWidget.DrawWindowBackground | QWidget.DrawChildren))) None
- render(self, target: PySide2.QtGui.QPaintDevice, targetOffset: PySide2.QtCore.QPoint = Default(QPoint), sourceRegion: PySide2.QtGui.QRegion = Default(QRegion), renderFlags: PySide2.QtWidgets.QWidget.RenderFlags = Instance(QWidget.RenderFlags(QWidget.DrawWindowBackground | QWidget.DrawChildren))) None
- repaint(self) None
- repaint(self, arg__1: PySide2.QtCore.QRect) None
- repaint(self, arg__1: PySide2.QtGui.QRegion) None
- repaint(self, x: int, y: int, w: int, h: int) None
- saveGeometry(self) PySide2.QtCore.QByteArray
- screen(self) PySide2.QtGui.QScreen
- scroll(self, dx: int, dy: int) None
- scroll(self, dx: int, dy: int, arg__3: PySide2.QtCore.QRect) None
- sender(self) PySide2.QtCore.QObject
- setBaseSize(self, arg__1: PySide2.QtCore.QSize) None
- setBaseSize(self, basew: int, baseh: int) None
- setContentsMargins(self, left: int, top: int, right: int, bottom: int) None
- setContentsMargins(self, margins: PySide2.QtCore.QMargins) None
- setGeometry(self, arg__1: PySide2.QtCore.QRect) None
- setGeometry(self, x: int, y: int, w: int, h: int) None
- setMask(self, arg__1: PySide2.QtGui.QBitmap) None
- setMask(self, arg__1: PySide2.QtGui.QRegion) None
- setMaximumSize(self, arg__1: PySide2.QtCore.QSize) None
- setMaximumSize(self, maxw: int, maxh: int) None
- setMinimumSize(self, arg__1: PySide2.QtCore.QSize) None
- setMinimumSize(self, minw: int, minh: int) None
- setParent(self, parent: PySide2.QtCore.QObject) None
- setParent(self, parent: PySide2.QtWidgets.QWidget) None
- setParent(self, parent: PySide2.QtWidgets.QWidget, f: PySide2.QtCore.Qt.WindowFlags) None
- setSizeIncrement(self, arg__1: PySide2.QtCore.QSize) None
- setSizeIncrement(self, w: int, h: int) None
- setSizePolicy(self, arg__1: PySide2.QtWidgets.QSizePolicy) None
- setSizePolicy(self, horizontal: PySide2.QtWidgets.QSizePolicy.Policy, vertical: PySide2.QtWidgets.QSizePolicy.Policy) None
- size(self) PySide2.QtCore.QSize
- sizeHint(self) PySide2.QtCore.QSize
- sizeIncrement(self) PySide2.QtCore.QSize
- sizePolicy(self) PySide2.QtWidgets.QSizePolicy
- startTimer(self, interval: int, timerType: PySide2.QtCore.Qt.TimerType = PySide2.QtCore.Qt.TimerType.CoarseTimer) int
- style(self) PySide2.QtWidgets.QStyle
- textFormat(self) PySide2.QtCore.Qt.TextFormat
- textInteractionFlags(self) PySide2.QtCore.Qt.TextInteractionFlags
- thread(self) PySide2.QtCore.QThread
- topLevelWidget(self) PySide2.QtWidgets.QWidget
- update(self) None
- update(self, arg__1: PySide2.QtCore.QRect) None
- update(self, arg__1: PySide2.QtGui.QRegion) None
- update(self, x: int, y: int, w: int, h: int) None
- visibleRegion(self) PySide2.QtGui.QRegion
- window(self) PySide2.QtWidgets.QWidget
- windowFlags(self) PySide2.QtCore.Qt.WindowFlags
- windowHandle(self) PySide2.QtGui.QWindow
- windowIcon(self) PySide2.QtGui.QIcon
- windowModality(self) PySide2.QtCore.Qt.WindowModality
- windowState(self) PySide2.QtCore.Qt.WindowStates
- windowType(self) PySide2.QtCore.Qt.WindowType
ShotgunModelOverlayWidget
- class overlay_widget.ShotgunModelOverlayWidget(sg_model, parent=None)[source]
Bases:
ShotgunOverlayWidget
A convenience class specifically designed to work with a
ShotgunModel
.By using this class, multiple overlay widgets can be easily created and connected to the same shotgun model.
- Parameters:
sg_model (
ShotgunModel
) – Shotgun model that this widget should connect toparent (
PySide.QtGui.QWidget
) – Widget to attach the overlay to
- set_model(sg_model)[source]
Set the model this widget should be connected to
- Parameters:
sg_model (
ShotgunModel
) – Shotgun model that this widget should connect to