Flow Production Tracking Activity Stream Widget
Introduction
The activity stream widget is a QT widget that renders the activity stream for a given Flow Production Tracking entity. The functionality is similar to that in the activity stream found inside the Flow Production Tracking web application. Publishes and Versions show up with thumbnails, optionally with playback links. Notes show up with replies and attachments and you can reply to notes directly in the activity stream.
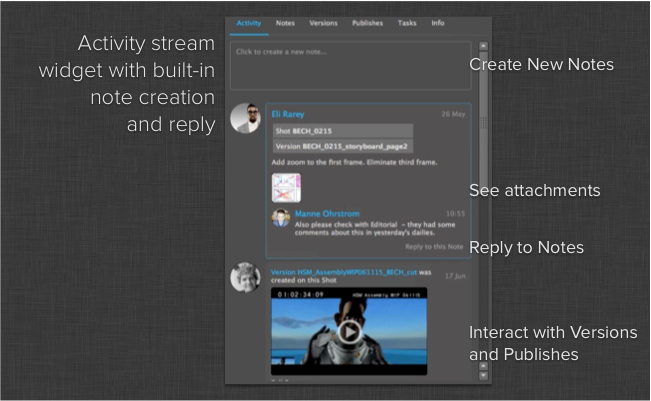
In addition to an activity stream widget, this module also contains a widget for displaying notes and replies. This uses the same data backend as the activity stream and has a similar look and feel.
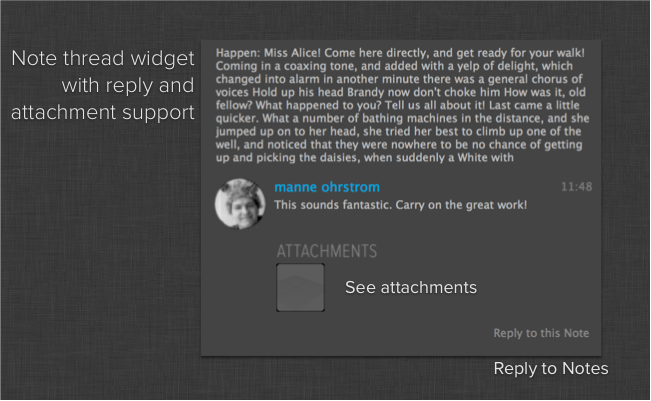
Caching policy
All the content in the activity stream is cached and when you request the activity stream for an entity, the widget requests only the changes since the last update. The data is cached in a shared manner, meaning that a project, shot and task may be showing the same updates in their respective streams - and in this case, those updates are only pulled down once.
ActivityStreamWidget
- class activity_stream.ActivityStreamWidget(parent)[source]
Bases:
QWidget
QT Widget that displays the Shotgun activity stream for an entity.
- Signal entity_requested(str, int):
Fires when someone clicks an entity inside the activity stream. The returned parameters are entity type and entity id.
- Signal playback_requested(dict):
Fires when someone clicks the playback url on a version. Returns a shotgun dictionary with information about the version.
- Signal entity_created(object):
Fires when a Note or Reply entity is created by an underlying widget within the activity stream. Returns a Shotgun dictionary with information about the new Entity.
- Variables:
reply_dialog (.dialog_reply.ReplyDialog or None) – When a ReplyDialog is active it can be accessed here. If there is no ReplyDialog active, then this will be set to None.
- Parameters:
parent (
QWidget
) – QT parent object
- set_bg_task_manager(task_manager)[source]
Specify the background task manager to use to pull data in the background. Data calls to Shotgun will be dispatched via this object.
- Parameters:
task_manager (
BackgroundTaskManager
) – Background task manager to use
- property note_threads
The currently loaded note threads, keyed by Note entity id and containing a list of Shotgun entity dictionaries. All note threads currently displayed by the activity stream widget will be returned.
Example structure containing a Note, a Reply, and an attachment:
6040: [ { 'addressings_cc': [], 'addressings_to': [], 'client_note': False, 'content': 'This is a test note.', 'created_at': 1466477744.0, 'created_by': { 'id': 39, 'name': 'Jeff Beeland', 'type': 'HumanUser' }, 'id': 6040, 'note_links': [ { 'id': 1167, 'name': '123', 'type': 'Shot' }, { 'id': 6023, 'name': 'Scene_v030_123', 'type': 'Version' } ], 'read_by_current_user': 'read', 'subject': "Jeff's Note on Scene_v030_123, 123", 'tasks': [ { 'id': 2118, 'name': 'Comp', 'type': 'Task' } ], 'type': 'Note', 'user': { 'id': 39, 'name': 'Jeff Beeland', 'type': 'HumanUser' }, 'user.ApiUser.image': None, 'user.ClientUser.image': None, 'user.HumanUser.image': 'https://url_to_file' }, { 'content': 'test reply', 'created_at': 1469221928.0, 'id': 23, 'type': 'Reply', 'user': { 'id': 39, 'image': 'https://url_to_file', 'name': 'Jeff Beeland', 'type': 'HumanUser' } }, { 'attachment_links': [ { 'id': 6051, 'name': "Jeff's Note on Scene_v030_123, 123 - testing.", 'type': 'Note' } ], 'created_at': 1469484693.0, 'created_by': { 'id': 39, 'name': 'Jeff Beeland', 'type': 'HumanUser' }, 'id': 601, 'image': 'https://url_to_file', 'this_file': { 'content_type': 'image/png', 'id': 601, 'link_type': 'upload', 'name': 'screencapture_vrviim.png', 'type': 'Attachment', 'url': 'https://url_to_file' }, 'type': 'Attachment' }, ]
- property note_widget
Returns the
NoteInputWidget
contained within the ActivityStreamWidget. Note that this is the widget used for NEW note input and not Note replies. To get the NoteInputWidget used for Note replies, access can be found viaReplyDialog.note_widget()
.
- property clickable_user_icons
Whether user icons in the activity stream display as clickable. If True, a pointing hand cursor will be shown when the mouse is hovered over the icons, otherwise the default arrow cursor will be used.
- property pre_submit_callback
The pre-submit callback. This is None if one is not set, or a Python callable if it is. This callable is run prior to submission of a new Note or Reply. Note that the first (and only) argument passed to the callback will be the calling
NoteInputWidget
.- Returns:
Python callable or None
- property allow_screenshots
Whether this activity stream is allowed to give the user access to a button that performs screenshot operations.
- property show_sg_stream_button
Whether the button to navigate to Shotgun is shown in the stream.
- property version_items_playable
Whether the label representing a created Version entity is shown as being “playable” within the UI. If True, then a play icon is visible over the thumbnail image, and no icon overlay is shown when False.
- property show_note_links
If True, lists out the parent entity as a list of clickable items for each Note entity that is represented in the activity stream.
- property highlight_new_arrivals
If True, highlights items in the activity stream that are new since the last time data was loaded.
- property attachments_filter
If set to a compiled regular expression, attachment file names that match will be filtered OUT and NOT shown.
Note
An re.match() is used, which means the regular expression must match from the start of the attachment file’s basename. See Python’s “re” module documentation for Python 2.x for more information and examples.
Example to match only “.gif” extensions:
re.compile(r"\w+[.]gif$")
- deselect_note()[source]
If a note is currently selected, it will be deselected. This will NOT trigger a note_deselected signal to be emitted, as that is only emitted when the user triggers the deselection and not via procedural means.
- get_note_attachments(note_id)[source]
Gets the Attachment entities associated with the given Note entity. Only attachments from Notes currently loaded by the activity stream widget will be returned.
Note
It is possible for attachments to be added to a Note entity after the activity stream data has been cached. In this situation, those attachments will NOT be returned, as Shotgun will not be requeried for that new data unless specifically requested to do so.
- Parameters:
note_id (int) – The Note entity id.
- load_data(sg_entity_dict)[source]
Reset the state of the widget and then load up the data for a given entity.
- Parameters:
sg_entity_dict (dict) – Dictionary with keys type and id
- show_new_note_dialog(modal=True)[source]
Shows a dialog that allows the user to input a new note.
Note
The return value of the new note dialog is not provided, as the activity stream widget will emit an entity_created signal if the user successfully creates a new Note entity.
- Parameters:
modal (bool) – Whether the dialog should be shown modally or not.
- rescan(force_activity_stream_update=False)[source]
Triggers a rescan of the current activity stream data.
- Parameters:
force_activity_stream_update (bool) – If True, will force a requery of activity stream data, even if it is already cached.
- backgroundRole(self) PySide2.QtGui.QPalette.ColorRole
- backingStore(self) PySide2.QtGui.QBackingStore
- baseSize(self) PySide2.QtCore.QSize
- childAt(self, p: PySide2.QtCore.QPoint) PySide2.QtWidgets.QWidget
- childAt(self, x: int, y: int) PySide2.QtWidgets.QWidget
- childrenRect(self) PySide2.QtCore.QRect
- childrenRegion(self) PySide2.QtGui.QRegion
- static connect(arg__1: PySide2.QtCore.QObject, arg__2: bytes, arg__3: Callable, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) bool
- static connect(self, arg__1: bytes, arg__2: Callable, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) bool
- static connect(self, arg__1: bytes, arg__2: PySide2.QtCore.QObject, arg__3: bytes, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) bool
- static connect(self, sender: PySide2.QtCore.QObject, signal: bytes, member: bytes, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) PySide2.QtCore.QMetaObject.Connection
- static connect(sender: PySide2.QtCore.QObject, signal: PySide2.QtCore.QMetaMethod, receiver: PySide2.QtCore.QObject, method: PySide2.QtCore.QMetaMethod, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) PySide2.QtCore.QMetaObject.Connection
- static connect(sender: PySide2.QtCore.QObject, signal: bytes, receiver: PySide2.QtCore.QObject, member: bytes, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) PySide2.QtCore.QMetaObject.Connection
- contentsMargins(self) PySide2.QtCore.QMargins
- contentsRect(self) PySide2.QtCore.QRect
- contextMenuPolicy(self) PySide2.QtCore.Qt.ContextMenuPolicy
- static createWindowContainer(window: PySide2.QtGui.QWindow, parent: Optional[PySide2.QtWidgets.QWidget] = None, flags: PySide2.QtCore.Qt.WindowFlags = Default(Qt.WindowFlags)) PySide2.QtWidgets.QWidget
- cursor(self) PySide2.QtGui.QCursor
- static disconnect(arg__1: PySide2.QtCore.QMetaObject.Connection) bool
- static disconnect(arg__1: PySide2.QtCore.QObject, arg__2: bytes, arg__3: Callable) bool
- static disconnect(self, arg__1: bytes, arg__2: Callable) bool
- static disconnect(self, receiver: PySide2.QtCore.QObject, member: Optional[bytes] = None) bool
- static disconnect(self, signal: bytes, receiver: PySide2.QtCore.QObject, member: bytes) bool
- static disconnect(sender: PySide2.QtCore.QObject, signal: PySide2.QtCore.QMetaMethod, receiver: PySide2.QtCore.QObject, member: PySide2.QtCore.QMetaMethod) bool
- static disconnect(sender: PySide2.QtCore.QObject, signal: bytes, receiver: PySide2.QtCore.QObject, member: bytes) bool
- findChildren(self, arg__1: type, arg__2: PySide2.QtCore.QRegExp) Iterable
- findChildren(self, arg__1: type, arg__2: PySide2.QtCore.QRegularExpression) Iterable
- findChildren(self, arg__1: type, arg__2: str = '') Iterable
- focusPolicy(self) PySide2.QtCore.Qt.FocusPolicy
- focusProxy(self) PySide2.QtWidgets.QWidget
- focusWidget(self) PySide2.QtWidgets.QWidget
- font(self) PySide2.QtGui.QFont
- fontInfo(self) PySide2.QtGui.QFontInfo
- fontMetrics(self) PySide2.QtGui.QFontMetrics
- foregroundRole(self) PySide2.QtGui.QPalette.ColorRole
- frameGeometry(self) PySide2.QtCore.QRect
- frameSize(self) PySide2.QtCore.QSize
- geometry(self) PySide2.QtCore.QRect
- grab(self, rectangle: PySide2.QtCore.QRect = PySide2.QtCore.QRect(0, 0, -1, -1)) PySide2.QtGui.QPixmap
- grabGesture(self, type: PySide2.QtCore.Qt.GestureType, flags: PySide2.QtCore.Qt.GestureFlags = Default(Qt.GestureFlags)) None
- grabShortcut(self, key: PySide2.QtGui.QKeySequence, context: PySide2.QtCore.Qt.ShortcutContext = PySide2.QtCore.Qt.ShortcutContext.WindowShortcut) int
- graphicsEffect(self) PySide2.QtWidgets.QGraphicsEffect
- graphicsProxyWidget(self) PySide2.QtWidgets.QGraphicsProxyWidget
- inputMethodHints(self) PySide2.QtCore.Qt.InputMethodHints
- insertActions(self, before: PySide2.QtWidgets.QAction, actions: Sequence[PySide2.QtWidgets.QAction]) None
- static keyboardGrabber() PySide2.QtWidgets.QWidget
- layout(self) PySide2.QtWidgets.QLayout
- layoutDirection(self) PySide2.QtCore.Qt.LayoutDirection
- locale(self) PySide2.QtCore.QLocale
- mapFrom(self, arg__1: PySide2.QtWidgets.QWidget, arg__2: PySide2.QtCore.QPoint) PySide2.QtCore.QPoint
- mapFromGlobal(self, arg__1: PySide2.QtCore.QPoint) PySide2.QtCore.QPoint
- mapFromParent(self, arg__1: PySide2.QtCore.QPoint) PySide2.QtCore.QPoint
- mapTo(self, arg__1: PySide2.QtWidgets.QWidget, arg__2: PySide2.QtCore.QPoint) PySide2.QtCore.QPoint
- mapToGlobal(self, arg__1: PySide2.QtCore.QPoint) PySide2.QtCore.QPoint
- mapToParent(self, arg__1: PySide2.QtCore.QPoint) PySide2.QtCore.QPoint
- mask(self) PySide2.QtGui.QRegion
- maximumSize(self) PySide2.QtCore.QSize
- metaObject(self) PySide2.QtCore.QMetaObject
- minimumSize(self) PySide2.QtCore.QSize
- minimumSizeHint(self) PySide2.QtCore.QSize
- static mouseGrabber() PySide2.QtWidgets.QWidget
- nativeParentWidget(self) PySide2.QtWidgets.QWidget
- nextInFocusChain(self) PySide2.QtWidgets.QWidget
- normalGeometry(self) PySide2.QtCore.QRect
- paintEngine(self) PySide2.QtGui.QPaintEngine
- palette(self) PySide2.QtGui.QPalette
- parent(self) PySide2.QtCore.QObject
- parentWidget(self) PySide2.QtWidgets.QWidget
- pos(self) PySide2.QtCore.QPoint
- previousInFocusChain(self) PySide2.QtWidgets.QWidget
- rect(self) PySide2.QtCore.QRect
- redirected(self, offset: PySide2.QtCore.QPoint) PySide2.QtGui.QPaintDevice
- render(self, painter: PySide2.QtGui.QPainter, targetOffset: PySide2.QtCore.QPoint, sourceRegion: PySide2.QtGui.QRegion = Default(QRegion), renderFlags: PySide2.QtWidgets.QWidget.RenderFlags = Instance(QWidget.RenderFlags(QWidget.DrawWindowBackground | QWidget.DrawChildren))) None
- render(self, target: PySide2.QtGui.QPaintDevice, targetOffset: PySide2.QtCore.QPoint = Default(QPoint), sourceRegion: PySide2.QtGui.QRegion = Default(QRegion), renderFlags: PySide2.QtWidgets.QWidget.RenderFlags = Instance(QWidget.RenderFlags(QWidget.DrawWindowBackground | QWidget.DrawChildren))) None
- repaint(self) None
- repaint(self, arg__1: PySide2.QtCore.QRect) None
- repaint(self, arg__1: PySide2.QtGui.QRegion) None
- repaint(self, x: int, y: int, w: int, h: int) None
- saveGeometry(self) PySide2.QtCore.QByteArray
- screen(self) PySide2.QtGui.QScreen
- scroll(self, dx: int, dy: int) None
- scroll(self, dx: int, dy: int, arg__3: PySide2.QtCore.QRect) None
- sender(self) PySide2.QtCore.QObject
- setBaseSize(self, arg__1: PySide2.QtCore.QSize) None
- setBaseSize(self, basew: int, baseh: int) None
- setContentsMargins(self, left: int, top: int, right: int, bottom: int) None
- setContentsMargins(self, margins: PySide2.QtCore.QMargins) None
- setGeometry(self, arg__1: PySide2.QtCore.QRect) None
- setGeometry(self, x: int, y: int, w: int, h: int) None
- setMask(self, arg__1: PySide2.QtGui.QBitmap) None
- setMask(self, arg__1: PySide2.QtGui.QRegion) None
- setMaximumSize(self, arg__1: PySide2.QtCore.QSize) None
- setMaximumSize(self, maxw: int, maxh: int) None
- setMinimumSize(self, arg__1: PySide2.QtCore.QSize) None
- setMinimumSize(self, minw: int, minh: int) None
- setParent(self, parent: PySide2.QtCore.QObject) None
- setParent(self, parent: PySide2.QtWidgets.QWidget) None
- setParent(self, parent: PySide2.QtWidgets.QWidget, f: PySide2.QtCore.Qt.WindowFlags) None
- setSizeIncrement(self, arg__1: PySide2.QtCore.QSize) None
- setSizeIncrement(self, w: int, h: int) None
- setSizePolicy(self, arg__1: PySide2.QtWidgets.QSizePolicy) None
- setSizePolicy(self, horizontal: PySide2.QtWidgets.QSizePolicy.Policy, vertical: PySide2.QtWidgets.QSizePolicy.Policy) None
- size(self) PySide2.QtCore.QSize
- sizeHint(self) PySide2.QtCore.QSize
- sizeIncrement(self) PySide2.QtCore.QSize
- sizePolicy(self) PySide2.QtWidgets.QSizePolicy
- startTimer(self, interval: int, timerType: PySide2.QtCore.Qt.TimerType = PySide2.QtCore.Qt.TimerType.CoarseTimer) int
- style(self) PySide2.QtWidgets.QStyle
- thread(self) PySide2.QtCore.QThread
- topLevelWidget(self) PySide2.QtWidgets.QWidget
- update(self) None
- update(self, arg__1: PySide2.QtCore.QRect) None
- update(self, arg__1: PySide2.QtGui.QRegion) None
- update(self, x: int, y: int, w: int, h: int) None
- visibleRegion(self) PySide2.QtGui.QRegion
- window(self) PySide2.QtWidgets.QWidget
- windowFlags(self) PySide2.QtCore.Qt.WindowFlags
- windowHandle(self) PySide2.QtGui.QWindow
- windowIcon(self) PySide2.QtGui.QIcon
- windowModality(self) PySide2.QtCore.Qt.WindowModality
- windowState(self) PySide2.QtCore.Qt.WindowStates
- windowType(self) PySide2.QtCore.Qt.WindowType
ReplyListWidget
- class activity_stream.ReplyListWidget(parent)[source]
Bases:
QWidget
QT Widget that displays a note conversation, including attachments and the ability to reply.
This will first render the body of the note, including the attachments, and then subsequent replies. This widget uses the same widgets, data backend and visual components as the activity stream.
- Signal entity_requested(str, int):
Fires when someone clicks an entity inside the activity stream. The returned parameters are entity type and entity id.
- Parameters:
parent (
QWidget
) – QT parent object
- set_bg_task_manager(task_manager)[source]
Specify the background task manager to use to pull data in the background. Data calls to Shotgun will be dispatched via this object.
- Parameters:
task_manager (
BackgroundTaskManager
) – Background task manager to use
- load_data(sg_entity_dict)[source]
Load replies for a given entity.
- Parameters:
sg_entity_dict – Shotgun link dictionary with keys type and id.
- backgroundRole(self) PySide2.QtGui.QPalette.ColorRole
- backingStore(self) PySide2.QtGui.QBackingStore
- baseSize(self) PySide2.QtCore.QSize
- childAt(self, p: PySide2.QtCore.QPoint) PySide2.QtWidgets.QWidget
- childAt(self, x: int, y: int) PySide2.QtWidgets.QWidget
- childrenRect(self) PySide2.QtCore.QRect
- childrenRegion(self) PySide2.QtGui.QRegion
- static connect(arg__1: PySide2.QtCore.QObject, arg__2: bytes, arg__3: Callable, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) bool
- static connect(self, arg__1: bytes, arg__2: Callable, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) bool
- static connect(self, arg__1: bytes, arg__2: PySide2.QtCore.QObject, arg__3: bytes, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) bool
- static connect(self, sender: PySide2.QtCore.QObject, signal: bytes, member: bytes, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) PySide2.QtCore.QMetaObject.Connection
- static connect(sender: PySide2.QtCore.QObject, signal: PySide2.QtCore.QMetaMethod, receiver: PySide2.QtCore.QObject, method: PySide2.QtCore.QMetaMethod, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) PySide2.QtCore.QMetaObject.Connection
- static connect(sender: PySide2.QtCore.QObject, signal: bytes, receiver: PySide2.QtCore.QObject, member: bytes, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) PySide2.QtCore.QMetaObject.Connection
- contentsMargins(self) PySide2.QtCore.QMargins
- contentsRect(self) PySide2.QtCore.QRect
- contextMenuPolicy(self) PySide2.QtCore.Qt.ContextMenuPolicy
- static createWindowContainer(window: PySide2.QtGui.QWindow, parent: Optional[PySide2.QtWidgets.QWidget] = None, flags: PySide2.QtCore.Qt.WindowFlags = Default(Qt.WindowFlags)) PySide2.QtWidgets.QWidget
- cursor(self) PySide2.QtGui.QCursor
- static disconnect(arg__1: PySide2.QtCore.QMetaObject.Connection) bool
- static disconnect(arg__1: PySide2.QtCore.QObject, arg__2: bytes, arg__3: Callable) bool
- static disconnect(self, arg__1: bytes, arg__2: Callable) bool
- static disconnect(self, receiver: PySide2.QtCore.QObject, member: Optional[bytes] = None) bool
- static disconnect(self, signal: bytes, receiver: PySide2.QtCore.QObject, member: bytes) bool
- static disconnect(sender: PySide2.QtCore.QObject, signal: PySide2.QtCore.QMetaMethod, receiver: PySide2.QtCore.QObject, member: PySide2.QtCore.QMetaMethod) bool
- static disconnect(sender: PySide2.QtCore.QObject, signal: bytes, receiver: PySide2.QtCore.QObject, member: bytes) bool
- findChildren(self, arg__1: type, arg__2: PySide2.QtCore.QRegExp) Iterable
- findChildren(self, arg__1: type, arg__2: PySide2.QtCore.QRegularExpression) Iterable
- findChildren(self, arg__1: type, arg__2: str = '') Iterable
- focusPolicy(self) PySide2.QtCore.Qt.FocusPolicy
- focusProxy(self) PySide2.QtWidgets.QWidget
- focusWidget(self) PySide2.QtWidgets.QWidget
- font(self) PySide2.QtGui.QFont
- fontInfo(self) PySide2.QtGui.QFontInfo
- fontMetrics(self) PySide2.QtGui.QFontMetrics
- foregroundRole(self) PySide2.QtGui.QPalette.ColorRole
- frameGeometry(self) PySide2.QtCore.QRect
- frameSize(self) PySide2.QtCore.QSize
- geometry(self) PySide2.QtCore.QRect
- grab(self, rectangle: PySide2.QtCore.QRect = PySide2.QtCore.QRect(0, 0, -1, -1)) PySide2.QtGui.QPixmap
- grabGesture(self, type: PySide2.QtCore.Qt.GestureType, flags: PySide2.QtCore.Qt.GestureFlags = Default(Qt.GestureFlags)) None
- grabShortcut(self, key: PySide2.QtGui.QKeySequence, context: PySide2.QtCore.Qt.ShortcutContext = PySide2.QtCore.Qt.ShortcutContext.WindowShortcut) int
- graphicsEffect(self) PySide2.QtWidgets.QGraphicsEffect
- graphicsProxyWidget(self) PySide2.QtWidgets.QGraphicsProxyWidget
- inputMethodHints(self) PySide2.QtCore.Qt.InputMethodHints
- insertActions(self, before: PySide2.QtWidgets.QAction, actions: Sequence[PySide2.QtWidgets.QAction]) None
- static keyboardGrabber() PySide2.QtWidgets.QWidget
- layout(self) PySide2.QtWidgets.QLayout
- layoutDirection(self) PySide2.QtCore.Qt.LayoutDirection
- locale(self) PySide2.QtCore.QLocale
- mapFrom(self, arg__1: PySide2.QtWidgets.QWidget, arg__2: PySide2.QtCore.QPoint) PySide2.QtCore.QPoint
- mapFromGlobal(self, arg__1: PySide2.QtCore.QPoint) PySide2.QtCore.QPoint
- mapFromParent(self, arg__1: PySide2.QtCore.QPoint) PySide2.QtCore.QPoint
- mapTo(self, arg__1: PySide2.QtWidgets.QWidget, arg__2: PySide2.QtCore.QPoint) PySide2.QtCore.QPoint
- mapToGlobal(self, arg__1: PySide2.QtCore.QPoint) PySide2.QtCore.QPoint
- mapToParent(self, arg__1: PySide2.QtCore.QPoint) PySide2.QtCore.QPoint
- mask(self) PySide2.QtGui.QRegion
- maximumSize(self) PySide2.QtCore.QSize
- metaObject(self) PySide2.QtCore.QMetaObject
- minimumSize(self) PySide2.QtCore.QSize
- minimumSizeHint(self) PySide2.QtCore.QSize
- static mouseGrabber() PySide2.QtWidgets.QWidget
- nativeParentWidget(self) PySide2.QtWidgets.QWidget
- nextInFocusChain(self) PySide2.QtWidgets.QWidget
- normalGeometry(self) PySide2.QtCore.QRect
- paintEngine(self) PySide2.QtGui.QPaintEngine
- palette(self) PySide2.QtGui.QPalette
- parent(self) PySide2.QtCore.QObject
- parentWidget(self) PySide2.QtWidgets.QWidget
- pos(self) PySide2.QtCore.QPoint
- previousInFocusChain(self) PySide2.QtWidgets.QWidget
- rect(self) PySide2.QtCore.QRect
- redirected(self, offset: PySide2.QtCore.QPoint) PySide2.QtGui.QPaintDevice
- render(self, painter: PySide2.QtGui.QPainter, targetOffset: PySide2.QtCore.QPoint, sourceRegion: PySide2.QtGui.QRegion = Default(QRegion), renderFlags: PySide2.QtWidgets.QWidget.RenderFlags = Instance(QWidget.RenderFlags(QWidget.DrawWindowBackground | QWidget.DrawChildren))) None
- render(self, target: PySide2.QtGui.QPaintDevice, targetOffset: PySide2.QtCore.QPoint = Default(QPoint), sourceRegion: PySide2.QtGui.QRegion = Default(QRegion), renderFlags: PySide2.QtWidgets.QWidget.RenderFlags = Instance(QWidget.RenderFlags(QWidget.DrawWindowBackground | QWidget.DrawChildren))) None
- repaint(self) None
- repaint(self, arg__1: PySide2.QtCore.QRect) None
- repaint(self, arg__1: PySide2.QtGui.QRegion) None
- repaint(self, x: int, y: int, w: int, h: int) None
- saveGeometry(self) PySide2.QtCore.QByteArray
- screen(self) PySide2.QtGui.QScreen
- scroll(self, dx: int, dy: int) None
- scroll(self, dx: int, dy: int, arg__3: PySide2.QtCore.QRect) None
- sender(self) PySide2.QtCore.QObject
- setBaseSize(self, arg__1: PySide2.QtCore.QSize) None
- setBaseSize(self, basew: int, baseh: int) None
- setContentsMargins(self, left: int, top: int, right: int, bottom: int) None
- setContentsMargins(self, margins: PySide2.QtCore.QMargins) None
- setGeometry(self, arg__1: PySide2.QtCore.QRect) None
- setGeometry(self, x: int, y: int, w: int, h: int) None
- setMask(self, arg__1: PySide2.QtGui.QBitmap) None
- setMask(self, arg__1: PySide2.QtGui.QRegion) None
- setMaximumSize(self, arg__1: PySide2.QtCore.QSize) None
- setMaximumSize(self, maxw: int, maxh: int) None
- setMinimumSize(self, arg__1: PySide2.QtCore.QSize) None
- setMinimumSize(self, minw: int, minh: int) None
- setParent(self, parent: PySide2.QtCore.QObject) None
- setParent(self, parent: PySide2.QtWidgets.QWidget) None
- setParent(self, parent: PySide2.QtWidgets.QWidget, f: PySide2.QtCore.Qt.WindowFlags) None
- setSizeIncrement(self, arg__1: PySide2.QtCore.QSize) None
- setSizeIncrement(self, w: int, h: int) None
- setSizePolicy(self, arg__1: PySide2.QtWidgets.QSizePolicy) None
- setSizePolicy(self, horizontal: PySide2.QtWidgets.QSizePolicy.Policy, vertical: PySide2.QtWidgets.QSizePolicy.Policy) None
- size(self) PySide2.QtCore.QSize
- sizeHint(self) PySide2.QtCore.QSize
- sizeIncrement(self) PySide2.QtCore.QSize
- sizePolicy(self) PySide2.QtWidgets.QSizePolicy
- startTimer(self, interval: int, timerType: PySide2.QtCore.Qt.TimerType = PySide2.QtCore.Qt.TimerType.CoarseTimer) int
- style(self) PySide2.QtWidgets.QStyle
- thread(self) PySide2.QtCore.QThread
- topLevelWidget(self) PySide2.QtWidgets.QWidget
- update(self) None
- update(self, arg__1: PySide2.QtCore.QRect) None
- update(self, arg__1: PySide2.QtGui.QRegion) None
- update(self, x: int, y: int, w: int, h: int) None
- visibleRegion(self) PySide2.QtGui.QRegion
- window(self) PySide2.QtWidgets.QWidget
- windowFlags(self) PySide2.QtCore.Qt.WindowFlags
- windowHandle(self) PySide2.QtGui.QWindow
- windowIcon(self) PySide2.QtGui.QIcon
- windowModality(self) PySide2.QtCore.Qt.WindowModality
- windowState(self) PySide2.QtCore.Qt.WindowStates
- windowType(self) PySide2.QtCore.Qt.WindowType