View Related Classes
This module contains various classes related to QT’s MVC model with a focus on the view side of the data hierarchy.
QT Widget Delegate System
The widget delegates module makes it easy to create custom UI experiences that are using data from the Flow Production Tracking Model.
If you feel that the visuals that you get back from a standard
QTreeView
or QListView
are not sufficient for your needs,
these view utilities provide a collection of tools to help you quickly build
consistent and nice looking user QT Views. These are typically used in conjunction
with the ShotgunModel
but this is not a requirement.
The widget delegate helper classes makes it easy to connect a QWidget
of
your choosing with a QT View. The classes will use your specified
widget as a brush to paint the view is drawn and updated. This allows for full control
of the visual appearance of any view, yet retaining the higher performance
you get in the QT MVC compared to an approach where large widget hierarchies are built up.
QT allows for customization of cell contents inside of its standard view classes through the use of so called delegate classes (see http://qt-project.org/doc/qt-4.8/model-view-programming.html#delegate-classes), however this can be cumbersome and difficult to get right. In an attempt to simplify the process of view customization, the Flow Production Tracking Qt Widgets framework contains classes for making it easy to plugin in standard QT widgets and use these as “brushes” when QT is drawing its views. You can then quickly produce UI in for example the QT designer, hook up the data exchange between your model class and the widget in a delegate and quickly have some nice custom looking user interfaces!
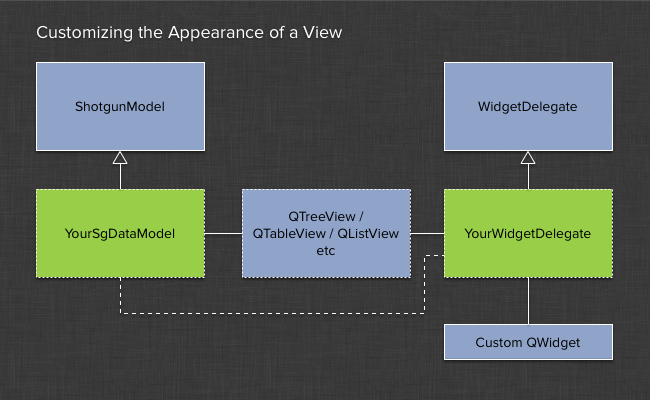
The principle is that you derive a custom delegate class from the WidgetDelegate
that is contained in the framework.
This class contains for simple methods that you need to implement. As part of this you can also control the flow of
data from the model into the widget each time it is being drawn, allowing for complex data to be easily passed from
Flow Production Tracking via the model into your widget.
The following example shows some of the basic around using this delegate class with the Flow Production Tracking Model:
# import the shotgun_model and view modules from the corresponding frameworks
shotgun_model = tank.platform.import_framework("tk-framework-shotgunutils", "shotgun_model")
shotgun_view = tank.platform.import_framework("tk-framework-qtwidgets", "views")
class ExampleDelegate(shotgun_view.WidgetDelegate):
def __init__(self, view):
"""
Constructor
"""
shotgun_view.WidgetDelegate.__init__(self, view)
def _create_widget(self, parent):
"""
Returns the widget to be used when drawing items
"""
# in this example, we use a simple QLabel to illustrate the principle.
# for more complex setups, you typically design a more complex widget
# and use this factory method to create it.
return QLabel(parent)
def _on_before_paint(self, widget, model_index, style_options):
"""
Called when a cell is about to be painted.
"""
# now we need to prepare our QLabel 'paintbrush' by setting
# some of the data members. The data is being provided from
# the underlying model via a model index pointer.
# note that the widget passed in is typically being reused
# across multiple cells in order to avoid creating lots of
# widget objects.
# get the Flow Production Tracking query data for this model item
sg_item = shotgun_model.get_sg_data(model_index)
# get the description
desc_str = sg_item.get("description")
# populate the QLabel with the description data
widget.setText(desc_str)
# QLabel is now ready to be used to paint this object on the screen
def _on_before_selection(self, widget, model_index, style_options):
"""
Called when a cell is being selected.
"""
# the widget object passed in here will not be used as a
# paintbrush but is a proper "live" object. This means
# that you can have buttons and other objects that a user
# can interact with as part of the widget. For items that
# are not selected, these buttons will merely be 'drawn'
# so they cannot for example exhibit any on-hover behavior.
# for selected objects however, the widget is live and
# can be interacted with. Therefore, the widget object
# in to this method is unique and will not be reused
# in other places.
# do std drawing first
self._on_before_paint(widget, model_index, style_options)
# at this point, we can indicate that the widget is
# selected, for example by setting the style
widget.setStyleSheet("{background-color: red;}")
def sizeHint(self, style_options, model_index):
"""
Base the size on the icon size property of the view
"""
# hint to the delegate system how much space our widget needs
QtCore.QSize(200, 100)
WidgetDelegate
- class views.WidgetDelegate(view)[source]
Bases:
QStyledItemDelegate
Convenience wrapper that makes it straight forward to use widgets inside of delegates.
This class is basically an adapter which lets you connect a view (
QAbstractItemView
) with aQWidget
of choice. This widget is used to “paint” the view when it is being rendered. When editing the item in the view, this class will create an editor widget as defined by the class.You use this class by subclassing it and implementing the methods:
_get_painter_widget()
- return the widget to be used to paint an index_on_before_paint()
- set up the widget with the specific data ready to be paintedsizeHint()
- return the size of the widget to be used in the view
If you want to provide an editor using the same widgetry then implement the following:
_create_editor_widget()
- return a unique editor instance to be used for editing the specific index - style options should be applied to the editor at this point.setEditorData()
- populate the editor with data from the modelsetModelData()
- apply the data from the editor back to the model
Note
If you are using the same widget for all items then you can just implement the
_create_widget()
method instead of the separate_get_painter_widget()
and_create_editor_widget()
methods.- Parameters:
view (
QWidget
) – The parent view for this delegate
- property view
Return the parent view of this delegate. This is just a wrapper for returning
self.parent()
but makes calling code easier to read!- Returns:
The parent view this delegate was created for
- Return type:
- _get_painter_widget(model_index, parent)[source]
Return a widget that can be used to paint the specified model index. If this is implemented in derived classes then the derived class is responsible for the lifetime of the widget.
- Parameters:
model_index (
QModelIndex
) – The index of the item in the model to return a widget forparent (
QWidget
) – The parent view that the widget should be parented to
- Returns:
A QWidget to be used for painting the current index
- Return type:
- _create_editor_widget(model_index, style_options, parent)[source]
Return a new editor widget for the specified model index. The base class is responsible for the lifetime of the widget meaning that the derived class should release all handles to it.
- Parameters:
model_index (
QModelIndex
) – The index of the item in the model to return a widget forstyle_options (
QStyleOptionViewItem
) – Specifies the current Qt style options for this indexparent (
QWidget
) – The parent view that the widget should be parented to
- Returns:
A QWidget to be used for editing the current index
- Return type:
- _on_before_paint(widget, model_index, style_options)[source]
This needs to be implemented by any deriving classes.
This is called just before a cell is painted. This method should configure values on the widget (such as labels, thumbnails etc) based on the data contained in the model index parameter which is being passed.
- Parameters:
widget – The QWidget (constructed in _create_widget()) which will be used to paint the cell.
model_index (
QModelIndex
) – object representing the data of the object that is about to be drawn.style_options (
QStyleOptionViewItem
) – Object containing specifics about the view related state of the cell.
- _create_widget(parent)[source]
This needs to be implemented by any deriving classes unless the separate methods
_get_painter_widget()
and_create_editor_widget()
are implemented instead.
EditSelectedWidgetDelegate
- class views.EditSelectedWidgetDelegate(view)[source]
Bases:
WidgetDelegate
Custom delegate that provides a simple mechanism where an actual widget (editor) is presented for the selected item whilst all other items are simply drawn with a single widget.
- Variables:
selection_model (QtGui.QItemSelectionModel) – The selection model of the delegate’s parent view, if one existed at the time of the delegate’s initialization.
You use this class by subclassing it and implementing the methods:
_get_painter_widget()
- return the widget to be used to paint an index_on_before_paint()
- set up the widget with the specific data ready to be paintedsizeHint()
- return the size of the widget to be used in the view
If you want to have an interactive widget (editor) for the selected item then you will also need to implement:
_create_editor_widget()
- return a unique editor instance to be used for editing_on_before_selection()
- set up the widget with the specific data ready for interaction
Note
If you are using the same widget for all items then you can just implement the
_create_widget()
method instead of the separate_get_painter_widget()
and_create_editor_widget()
methods.Note
In order for this class to handle selection correctly, it needs to be attached to the view after the model has been attached. (This is to ensure that it is able to obtain the view’s selection model correctly.)
- Parameters:
view (
QWidget
) – The parent view for this delegate
- _on_before_selection(widget, model_index, style_options)[source]
This method is called just before a cell is selected. This method should configure values on the widget (such as labels, thumbnails etc) based on the data contained in the model index parameter which is being passed.
- Parameters:
widget – The QWidget (constructed in _create_widget()) which will be used to paint the cell.
model_index (
QModelIndex
) – QModelIndex object representing the data of the object that is about to be drawn.style_options (
QStyleOptionViewItem
) – object containing specifics about the view related state of the cell.
- static connect(arg__1: PySide2.QtCore.QObject, arg__2: bytes, arg__3: Callable, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) → bool
- static connect(self, arg__1: bytes, arg__2: Callable, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) → bool
- static connect(self, arg__1: bytes, arg__2: PySide2.QtCore.QObject, arg__3: bytes, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) → bool
- static connect(self, sender: PySide2.QtCore.QObject, signal: bytes, member: bytes, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) → PySide2.QtCore.QMetaObject.Connection
- static connect(sender: PySide2.QtCore.QObject, signal: PySide2.QtCore.QMetaMethod, receiver: PySide2.QtCore.QObject, method: PySide2.QtCore.QMetaMethod, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) → PySide2.QtCore.QMetaObject.Connection
- static connect(sender: PySide2.QtCore.QObject, signal: bytes, receiver: PySide2.QtCore.QObject, member: bytes, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) → PySide2.QtCore.QMetaObject.Connection
- static disconnect(arg__1: PySide2.QtCore.QMetaObject.Connection) → bool
- static disconnect(arg__1: PySide2.QtCore.QObject, arg__2: bytes, arg__3: Callable) → bool
- static disconnect(self, arg__1: bytes, arg__2: Callable) → bool
- static disconnect(self, receiver: PySide2.QtCore.QObject, member: Optional[bytes] = None) → bool
- static disconnect(self, signal: bytes, receiver: PySide2.QtCore.QObject, member: bytes) → bool
- static disconnect(sender: PySide2.QtCore.QObject, signal: PySide2.QtCore.QMetaMethod, receiver: PySide2.QtCore.QObject, member: PySide2.QtCore.QMetaMethod) → bool
- static disconnect(sender: PySide2.QtCore.QObject, signal: bytes, receiver: PySide2.QtCore.QObject, member: bytes) → bool
- editorEvent(self, event: PySide2.QtCore.QEvent, model: PySide2.QtCore.QAbstractItemModel, option: PySide2.QtWidgets.QStyleOptionViewItem, index: PySide2.QtCore.QModelIndex) → bool
- static elidedText(fontMetrics: PySide2.QtGui.QFontMetrics, width: int, mode: PySide2.QtCore.Qt.TextElideMode, text: str) → str
- findChildren(self, arg__1: type, arg__2: PySide2.QtCore.QRegExp) → Iterable
- findChildren(self, arg__1: type, arg__2: PySide2.QtCore.QRegularExpression) → Iterable
- findChildren(self, arg__1: type, arg__2: str = '') → Iterable
- helpEvent(self, event: PySide2.QtGui.QHelpEvent, view: PySide2.QtWidgets.QAbstractItemView, option: PySide2.QtWidgets.QStyleOptionViewItem, index: PySide2.QtCore.QModelIndex) → bool
- initStyleOption(self, option: PySide2.QtWidgets.QStyleOptionViewItem, index: PySide2.QtCore.QModelIndex) → None
- itemEditorFactory(self) → PySide2.QtWidgets.QItemEditorFactory
- metaObject(self) → PySide2.QtCore.QMetaObject
- parent(self) → PySide2.QtCore.QObject
- sender(self) → PySide2.QtCore.QObject
- setModelData(self, editor: PySide2.QtWidgets.QWidget, model: PySide2.QtCore.QAbstractItemModel, index: PySide2.QtCore.QModelIndex) → None
- sizeHint(self, option: PySide2.QtWidgets.QStyleOptionViewItem, index: PySide2.QtCore.QModelIndex) → PySide2.QtCore.QSize
- startTimer(self, interval: int, timerType: PySide2.QtCore.Qt.TimerType = PySide2.QtCore.Qt.TimerType.CoarseTimer) → int
- thread(self) → PySide2.QtCore.QThread
Grouped List View
The grouped list view offers a visual representation where items are grouped into collapsible sections.
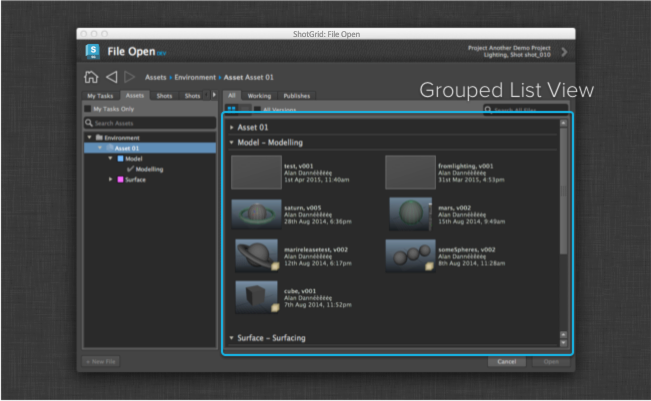
The grouped list view uses the WidgetDelegate
system in order to render
the UI. A custom QT view - GroupedListView
- is the main component. The view
expects a tree-like model structure with two levels, a grouping level and a data level.
When the data is loaded in, it will use a special delegate to render the grouping level of the tree structure. You can customize this delegate easily by providing your own widget. This widget needs to be able to react to expansion signals sent from the model so that it can change its appearance when the view decides to expand and contract the section.
The grouping delegate is typically designed as a header-like object. It shouldn’t contain any of its child items but rather act as a partition between groups. The collapse/expanded state can for example be indicated by an arrow that is either pointing down or sideways. The main view logic will take care of hiding any child objects.
If you want to create your own appearance, you can follow these rough steps:
First, design a grouping widget and make sure it derives from
GroupWidgetBase
.Make sure it implements the
GroupWidgetBase.set_expanded()
for handling its expanded state andGroupWidgetBase.set_item()
to receive data from the model about what text to display etc.Now, derive from the
GroupedListViewItemDelegate
class and implement the factory methodGroupedListViewItemDelegate.create_group_widget()
to return your custom grouping widget.If you want the child items to be rendered via custom widget delegates, you can modify your derived class at this point to do that too.
Lastly, pass your delegate to the view via the standard QT
PySide.QtGui.QAbstractItemView.setItemDelegate()
call to bind it to the view.
GroupedListView
- class views.GroupedListView(parent)[source]
Bases:
QAbstractItemView
Custom Qt View that displays items as a grouped list. The view works with any tree based model with the first level of the hierarchy defining the groups and the second level defining the items for that group. Subsequent levels of the hierarchy are ignored.
Items within a group are layed out left-to right and wrap automatically based on the view’s width.
For example, the following tree model:
- Group 1 - Item 1 - Item 2 - Item 3 - Group 2 - Item 4 - Group 3
Would look like this in the view:
> Group 1 ----------------- [Item 1] [Item 2] [Item 3] > Group 2 ----------------- [Item 4] > Group 3 -----------------
The widgets used for the various groups and items are created through a GroupedListViewItemDelegate and this can be overriden to implement custom UI for these elements.
- Parameters:
parent (
QWidget
) – The parent QWidget
- property border
The external border to use for all items in the view
- property group_spacing
The spacing to use between groups when they are collapsed
- property item_spacing
The spacing to use between items in the view
- expand(index)[source]
Expand the specified index
- Parameters:
index (
QModelIndex
) – The model index to be expanded
- collapse(index)[source]
Collapse the specified index
- Parameters:
index (
QModelIndex
) – The model index to be collapsed
- is_expanded(index)[source]
Query if the specified index is expanded or not
- Parameters:
index (
QModelIndex
) – The model index to check- Returns:
True if the index is a root index and is expanded, otherwise False
GroupedListViewItemDelegate
- class views.GroupedListViewItemDelegate(view)[source]
Bases:
WidgetDelegate
Base delegate class for a delegate specifically to be used by a
GroupedListView
.The delegate provides a method to return a group widget in addition to the regular delegate methods.
- Parameters:
view (
QWidget
) – The view this delegate is operating on
GroupWidgetBase
- class views.GroupWidgetBase[source]
Bases:
QWidget
Base interface for a group widget that will be used in the
GroupedListView
custom view- Signal toggle_expanded(bool):
Emitted when the group’s expansion state is toggled. Includes a boolean to indicate if the group is expanded or not.
- set_item(model_idx)[source]
Set the item this widget should be associated with. This should be implemented in derived classes
- Parameters:
model_idx – The index of the item in the model
- set_expanded(expand=True)[source]
Set if this widget is expanded or not. This should be implemented in derived classes
- Parameters:
expand (bool) – True if the widget should be expanded, False if it should be collapsed.
- backgroundRole(self) → PySide2.QtGui.QPalette.ColorRole
- backingStore(self) → PySide2.QtGui.QBackingStore
- baseSize(self) → PySide2.QtCore.QSize
- childAt(self, p: PySide2.QtCore.QPoint) → PySide2.QtWidgets.QWidget
- childAt(self, x: int, y: int) → PySide2.QtWidgets.QWidget
- childrenRect(self) → PySide2.QtCore.QRect
- childrenRegion(self) → PySide2.QtGui.QRegion
- static connect(arg__1: PySide2.QtCore.QObject, arg__2: bytes, arg__3: Callable, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) → bool
- static connect(self, arg__1: bytes, arg__2: Callable, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) → bool
- static connect(self, arg__1: bytes, arg__2: PySide2.QtCore.QObject, arg__3: bytes, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) → bool
- static connect(self, sender: PySide2.QtCore.QObject, signal: bytes, member: bytes, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) → PySide2.QtCore.QMetaObject.Connection
- static connect(sender: PySide2.QtCore.QObject, signal: PySide2.QtCore.QMetaMethod, receiver: PySide2.QtCore.QObject, method: PySide2.QtCore.QMetaMethod, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) → PySide2.QtCore.QMetaObject.Connection
- static connect(sender: PySide2.QtCore.QObject, signal: bytes, receiver: PySide2.QtCore.QObject, member: bytes, type: PySide2.QtCore.Qt.ConnectionType = PySide2.QtCore.Qt.ConnectionType.AutoConnection) → PySide2.QtCore.QMetaObject.Connection
- contentsMargins(self) → PySide2.QtCore.QMargins
- contentsRect(self) → PySide2.QtCore.QRect
- contextMenuPolicy(self) → PySide2.QtCore.Qt.ContextMenuPolicy
- static createWindowContainer(window: PySide2.QtGui.QWindow, parent: Optional[PySide2.QtWidgets.QWidget] = None, flags: PySide2.QtCore.Qt.WindowFlags = Default(Qt.WindowFlags)) → PySide2.QtWidgets.QWidget
- cursor(self) → PySide2.QtGui.QCursor
- static disconnect(arg__1: PySide2.QtCore.QMetaObject.Connection) → bool
- static disconnect(arg__1: PySide2.QtCore.QObject, arg__2: bytes, arg__3: Callable) → bool
- static disconnect(self, arg__1: bytes, arg__2: Callable) → bool
- static disconnect(self, receiver: PySide2.QtCore.QObject, member: Optional[bytes] = None) → bool
- static disconnect(self, signal: bytes, receiver: PySide2.QtCore.QObject, member: bytes) → bool
- static disconnect(sender: PySide2.QtCore.QObject, signal: PySide2.QtCore.QMetaMethod, receiver: PySide2.QtCore.QObject, member: PySide2.QtCore.QMetaMethod) → bool
- static disconnect(sender: PySide2.QtCore.QObject, signal: bytes, receiver: PySide2.QtCore.QObject, member: bytes) → bool
- findChildren(self, arg__1: type, arg__2: PySide2.QtCore.QRegExp) → Iterable
- findChildren(self, arg__1: type, arg__2: PySide2.QtCore.QRegularExpression) → Iterable
- findChildren(self, arg__1: type, arg__2: str = '') → Iterable
- focusPolicy(self) → PySide2.QtCore.Qt.FocusPolicy
- focusProxy(self) → PySide2.QtWidgets.QWidget
- focusWidget(self) → PySide2.QtWidgets.QWidget
- font(self) → PySide2.QtGui.QFont
- fontInfo(self) → PySide2.QtGui.QFontInfo
- fontMetrics(self) → PySide2.QtGui.QFontMetrics
- foregroundRole(self) → PySide2.QtGui.QPalette.ColorRole
- frameGeometry(self) → PySide2.QtCore.QRect
- frameSize(self) → PySide2.QtCore.QSize
- geometry(self) → PySide2.QtCore.QRect
- grab(self, rectangle: PySide2.QtCore.QRect = PySide2.QtCore.QRect(0, 0, -1, -1)) → PySide2.QtGui.QPixmap
- grabGesture(self, type: PySide2.QtCore.Qt.GestureType, flags: PySide2.QtCore.Qt.GestureFlags = Default(Qt.GestureFlags)) → None
- grabShortcut(self, key: PySide2.QtGui.QKeySequence, context: PySide2.QtCore.Qt.ShortcutContext = PySide2.QtCore.Qt.ShortcutContext.WindowShortcut) → int
- graphicsEffect(self) → PySide2.QtWidgets.QGraphicsEffect
- graphicsProxyWidget(self) → PySide2.QtWidgets.QGraphicsProxyWidget
- inputMethodHints(self) → PySide2.QtCore.Qt.InputMethodHints
- insertActions(self, before: PySide2.QtWidgets.QAction, actions: Sequence[PySide2.QtWidgets.QAction]) → None
- static keyboardGrabber() → PySide2.QtWidgets.QWidget
- layout(self) → PySide2.QtWidgets.QLayout
- layoutDirection(self) → PySide2.QtCore.Qt.LayoutDirection
- locale(self) → PySide2.QtCore.QLocale
- mapFrom(self, arg__1: PySide2.QtWidgets.QWidget, arg__2: PySide2.QtCore.QPoint) → PySide2.QtCore.QPoint
- mapFromGlobal(self, arg__1: PySide2.QtCore.QPoint) → PySide2.QtCore.QPoint
- mapFromParent(self, arg__1: PySide2.QtCore.QPoint) → PySide2.QtCore.QPoint
- mapTo(self, arg__1: PySide2.QtWidgets.QWidget, arg__2: PySide2.QtCore.QPoint) → PySide2.QtCore.QPoint
- mapToGlobal(self, arg__1: PySide2.QtCore.QPoint) → PySide2.QtCore.QPoint
- mapToParent(self, arg__1: PySide2.QtCore.QPoint) → PySide2.QtCore.QPoint
- mask(self) → PySide2.QtGui.QRegion
- maximumSize(self) → PySide2.QtCore.QSize
- metaObject(self) → PySide2.QtCore.QMetaObject
- minimumSize(self) → PySide2.QtCore.QSize
- minimumSizeHint(self) → PySide2.QtCore.QSize
- static mouseGrabber() → PySide2.QtWidgets.QWidget
- nativeParentWidget(self) → PySide2.QtWidgets.QWidget
- nextInFocusChain(self) → PySide2.QtWidgets.QWidget
- normalGeometry(self) → PySide2.QtCore.QRect
- paintEngine(self) → PySide2.QtGui.QPaintEngine
- palette(self) → PySide2.QtGui.QPalette
- parent(self) → PySide2.QtCore.QObject
- parentWidget(self) → PySide2.QtWidgets.QWidget
- pos(self) → PySide2.QtCore.QPoint
- previousInFocusChain(self) → PySide2.QtWidgets.QWidget
- rect(self) → PySide2.QtCore.QRect
- redirected(self, offset: PySide2.QtCore.QPoint) → PySide2.QtGui.QPaintDevice
- render(self, painter: PySide2.QtGui.QPainter, targetOffset: PySide2.QtCore.QPoint, sourceRegion: PySide2.QtGui.QRegion = Default(QRegion), renderFlags: PySide2.QtWidgets.QWidget.RenderFlags = Instance(QWidget.RenderFlags(QWidget.DrawWindowBackground | QWidget.DrawChildren))) → None
- render(self, target: PySide2.QtGui.QPaintDevice, targetOffset: PySide2.QtCore.QPoint = Default(QPoint), sourceRegion: PySide2.QtGui.QRegion = Default(QRegion), renderFlags: PySide2.QtWidgets.QWidget.RenderFlags = Instance(QWidget.RenderFlags(QWidget.DrawWindowBackground | QWidget.DrawChildren))) → None
- repaint(self) → None
- repaint(self, arg__1: PySide2.QtCore.QRect) → None
- repaint(self, arg__1: PySide2.QtGui.QRegion) → None
- repaint(self, x: int, y: int, w: int, h: int) → None
- saveGeometry(self) → PySide2.QtCore.QByteArray
- screen(self) → PySide2.QtGui.QScreen
- scroll(self, dx: int, dy: int) → None
- scroll(self, dx: int, dy: int, arg__3: PySide2.QtCore.QRect) → None
- sender(self) → PySide2.QtCore.QObject
- setBaseSize(self, arg__1: PySide2.QtCore.QSize) → None
- setBaseSize(self, basew: int, baseh: int) → None
- setContentsMargins(self, left: int, top: int, right: int, bottom: int) → None
- setContentsMargins(self, margins: PySide2.QtCore.QMargins) → None
- setGeometry(self, arg__1: PySide2.QtCore.QRect) → None
- setGeometry(self, x: int, y: int, w: int, h: int) → None
- setMask(self, arg__1: PySide2.QtGui.QBitmap) → None
- setMask(self, arg__1: PySide2.QtGui.QRegion) → None
- setMaximumSize(self, arg__1: PySide2.QtCore.QSize) → None
- setMaximumSize(self, maxw: int, maxh: int) → None
- setMinimumSize(self, arg__1: PySide2.QtCore.QSize) → None
- setMinimumSize(self, minw: int, minh: int) → None
- setParent(self, parent: PySide2.QtCore.QObject) → None
- setParent(self, parent: PySide2.QtWidgets.QWidget) → None
- setParent(self, parent: PySide2.QtWidgets.QWidget, f: PySide2.QtCore.Qt.WindowFlags) → None
- setSizeIncrement(self, arg__1: PySide2.QtCore.QSize) → None
- setSizeIncrement(self, w: int, h: int) → None
- setSizePolicy(self, arg__1: PySide2.QtWidgets.QSizePolicy) → None
- setSizePolicy(self, horizontal: PySide2.QtWidgets.QSizePolicy.Policy, vertical: PySide2.QtWidgets.QSizePolicy.Policy) → None
- sharedPainter(self) → PySide2.QtGui.QPainter
- size(self) → PySide2.QtCore.QSize
- sizeHint(self) → PySide2.QtCore.QSize
- sizeIncrement(self) → PySide2.QtCore.QSize
- sizePolicy(self) → PySide2.QtWidgets.QSizePolicy
- startTimer(self, interval: int, timerType: PySide2.QtCore.Qt.TimerType = PySide2.QtCore.Qt.TimerType.CoarseTimer) → int
- style(self) → PySide2.QtWidgets.QStyle
- thread(self) → PySide2.QtCore.QThread
- topLevelWidget(self) → PySide2.QtWidgets.QWidget
- update(self) → None
- update(self, arg__1: PySide2.QtCore.QRect) → None
- update(self, arg__1: PySide2.QtGui.QRegion) → None
- update(self, x: int, y: int, w: int, h: int) → None
- visibleRegion(self) → PySide2.QtGui.QRegion
- window(self) → PySide2.QtWidgets.QWidget
- windowFlags(self) → PySide2.QtCore.Qt.WindowFlags
- windowHandle(self) → PySide2.QtGui.QWindow
- windowIcon(self) → PySide2.QtGui.QIcon
- windowModality(self) → PySide2.QtCore.Qt.WindowModality
- windowState(self) → PySide2.QtCore.Qt.WindowStates
- windowType(self) → PySide2.QtCore.Qt.WindowType